Create Online Examination System using Java
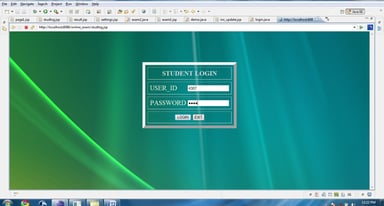
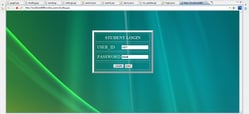
Creating an Online Examination System using Java involves both the frontend (user interface) and backend (handling the logic, database, and storing questions, user responses). Below is a simple example of how to create such a system using Java with basic features like multiple-choice questions, answering, and displaying results.
This example will include:
A simple Console-based interface.
A set of predefined questions.
Logic to submit answers and calculate scores.
Key Components:
Question Class: To represent each question and options.
Exam Class: To run the exam.
Main Class: To handle user interaction.
Steps:
Define the Question Model: This class will hold the question, options, and the correct answer.
Implement the Exam Logic: Handle asking the questions and checking answers.
Display the Score: After completing the exam, calculate the score and display the result.
Latest Posts
Quick Links
Create Online Examination System using Java
Follow Us
Explanation:
Question Class:
Each Question object contains the question text, an array of options, and the index of the correct answer.
It has a method isCorrect(int answer) to check if the selected answer is correct.
Exam Class:
The Exam class takes an array of Question objects.
The method startExam() displays each question with its options, accepts the user's input, checks if the answer is correct, and calculates the score.
OnlineExaminationSystem Class (Main Class):
In the main method, we create a few sample questions and store them in an array.
We create an Exam object with the set of questions and then start the exam by calling startExam(). After the exam ends, the score is displayed.
Running the System:
Add more questions: You can easily add more questions by creating new instances of the Question class and adding them to the array.
Input: The user can input their answer by choosing an option number (1, 2, 3, or 4).
Final Output:
Contact Info
© 2024. All rights reserved.
Navigate
Thanks 🙏 for visiting SimpleCode Join telegram (link available -Scroll Up) for source code files , pdf and
Any Promotion queries 👇